Cohere Embeddings
提供Bedrock Cohere Embedding客户端。将生成式AI功能集成到改善业务结果的基本应用程序和工作流程中。
AWS Bedrock Cohere模型页面和Amazon Bedrock用户指南包含有关如何使用AWS托管模型的详细信息。
前提条件
参考Spring AI文档关于Amazon Bedrock设置API访问。
自动配置
将spring-ai-bedrock-ai-spring-boot-starter
依赖添加到项目的Mavenpom.xml
文件中:
<dependency>
<groupId>org.springframework.ai</groupId>
<artifactId>spring-ai-bedrock-ai-spring-boot-starter</artifactId>
</dependency>
或者添加到Gradlebuild.gradle
构建文件中。
dependencies {
implementation 'org.springframework.ai:spring-ai-bedrock-ai-spring-boot-starter'
}
请参考依赖管理部分,将Spring AI BOM添加到您的构建文件中。 |
启用Cohere嵌入支持
默认情况下,Cohere模型被禁用。要启用它,请将spring.ai.bedrock.cohere.embedding.enabled
属性设置为true
。导出环境变量是设置此配置属性的一种方式:
export SPRING_AI_BEDROCK_COHERE_EMBEDDING_ENABLED=true
嵌入属性
前缀spring.ai.bedrock.aws
是配置连接到AWS Bedrock的属性前缀。
属性 | 描述 | 默认值 |
---|---|---|
spring.ai.bedrock.aws.region |
要使用的AWS区域。 |
us-east-1 |
spring.ai.bedrock.aws.access-key |
AWS访问密钥。 |
- |
spring.ai.bedrock.aws.secret-key |
AWS秘钥。 |
- |
前缀spring.ai.bedrock.cohere.embedding
(在BedrockCohereEmbeddingProperties
中定义)是配置Cohere的嵌入客户端实现的属性前缀。
属性 |
描述 |
默认值 |
spring.ai.bedrock.cohere.embedding.enabled |
启用或禁用Cohere支持 |
false |
spring.ai.bedrock.cohere.embedding.model |
要使用的模型ID。请参见支持的模型的CohereEmbeddingModel。 |
cohere.embed-multilingual-v3 |
spring.ai.bedrock.cohere.embedding.options.input-type |
在不同类型之间添加特殊标记以区分彼此。除了在搜索和检索时将不同类型混合在一起时,您不应该混合不同类型。在这种情况下,用search_document类型嵌入您的语料库,并使用type search_query嵌入查询。 |
SEARCH_DOCUMENT |
spring.ai.bedrock.cohere.embedding.options.truncate |
指定API如何处理长度超过最大标记长度的输入。如果指定LEFT或RIGHT,则模型丢弃输入,直到剩余输入恰好是模型的最大输入标记长度。 |
NONE |
查看其他模型ID,请访问CohereEmbeddingModel。支持的值有:cohere.embed-multilingual-v3
和 cohere.embed-english-v3
。模型ID值也可以在AWS Bedrock文档中的基本模型ID中找到。
所有以spring.ai.bedrock.cohere.embedding.options 为前缀的属性可以通过在EmbeddingRequest 调用中添加请求特定的嵌入选项来在运行时进行覆盖。 |
嵌入选项
BedrockCohereEmbeddingOptions.java 提供模型配置,例如 input-type
或 truncate
。
在启动时,可以使用 BedrockCohereEmbeddingClient(api, options)
构造函数或 spring.ai.bedrock.cohere.embedding.options.*
属性配置默认选项。
在运行时,您可以通过向 EmbeddingRequest
调用添加新的、特定于请求的选项来覆盖默认选项。例如,要覆盖特定请求的默认温度:
EmbeddingResponse embeddingResponse = embeddingClient.call(
new EmbeddingRequest(List.of("Hello World", "World is big and salvation is near"),
BedrockCohereEmbeddingOptions.builder()
.withInputType(InputType.SEARCH_DOCUMENT)
.build()));
示例控制器 (自动配置)
创建 一个新的 Spring Boot 项目并将 spring-ai-bedrock-ai-spring-boot-starter
添加到您的 pom(或 gradle)依赖项中。
在 src/main/resources
目录下添加一个 application.properties
文件,以启用和配置 Cohere Embedding 客户端:
spring.ai.bedrock.aws.region=eu-central-1
spring.ai.bedrock.aws.access-key=${AWS_ACCESS_KEY_ID}
spring.ai.bedrock.aws.secret-key=${AWS_SECRET_ACCESS_KEY}
spring.ai.bedrock.cohere.embedding.enabled=true
spring.ai.bedrock.cohere.embedding.options.input-type=search-document
用您的 AWS 凭证替换 regions 、access-key 和 secret-key 。 |
这将创建一个 BedrockCohereEmbeddingClient
实现,您可以将其注入到您的类中。这是一个简单的@Controller
类的示例,它使用聊天客户端进行文本生成。
@RestController
public class EmbeddingController {
private final EmbeddingClient embeddingClient;
@Autowired
public EmbeddingController(EmbeddingClient embeddingClient) {
this.embeddingClient = embeddingClient;
}
@GetMapping("/ai/embedding")
public Map embed(@RequestParam(value = "message", defaultValue = "Tell me a joke") String message) {
EmbeddingResponse embeddingResponse = this.embeddingClient.embedForResponse(List.of(message));
return Map.of("embedding", embeddingResponse);
}
}
手动配置
BedrockCohereEmbeddingClient实现了EmbeddingClient
接口,并使用低级别的CohereEmbeddingBedrockApi客户端连接到Bedrock Cohere服务。
在项目的Maven pom.xml
文件中添加spring-ai-bedrock
依赖:
<dependency>
<groupId>org.springframework.ai</groupId>
<artifactId>spring-ai-bedrock</artifactId>
</dependency>
或者添加到你的Gradle build.gradle
构建文件中。
dependencies {
implementation 'org.springframework.ai:spring-ai-bedrock'
}
请参考依赖管理部分,将Spring AI BOM添加到你的构建文件中。 |
接下来,创建一个BedrockCohereEmbeddingClient并用于文本嵌入:
var cohereEmbeddingApi =new CohereEmbeddingBedrockApi(
CohereEmbeddingModel.COHERE_EMBED_MULTILINGUAL_V1.id(),
EnvironmentVariableCredentialsProvider.create(), Region.US_EAST_1.id(), new ObjectMapper());
var embeddingClient = new BedrockCohereEmbeddingClient(cohereEmbeddingApi);
EmbeddingResponse embeddingResponse = embeddingClient
.embedForResponse(List.of("Hello World", "World is big and salvation is near"));
低级别CohereEmbeddingBedrockApi客户端
CohereEmbeddingBedrockApi是一个轻量级Java客户端,建立在AWS Bedrock Cohere命令模型之上。
下面的类图展示了CohereEmbeddingBedrockApi接口和构建块:
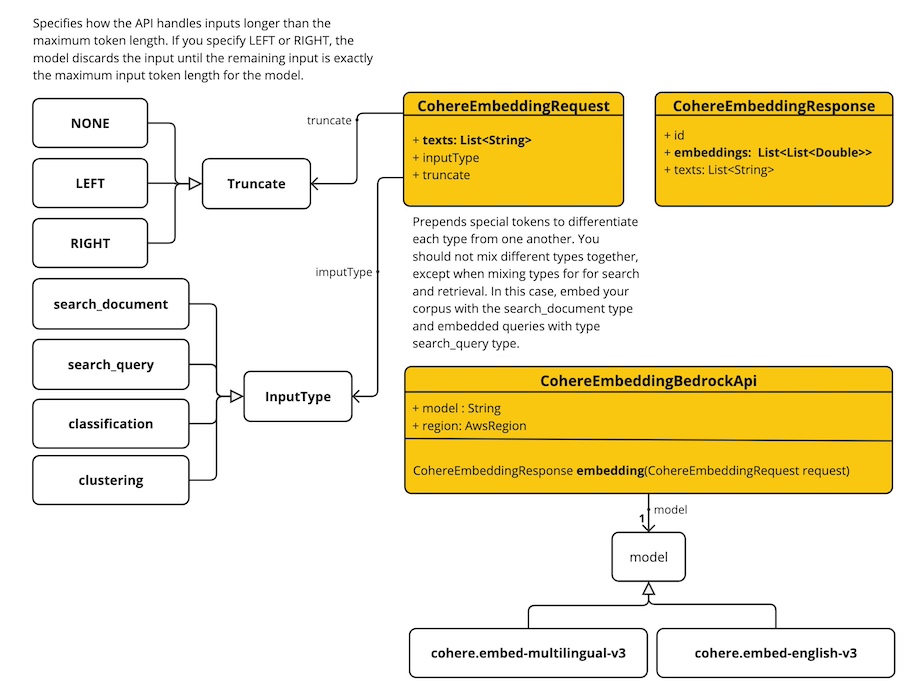
CohereEmbeddingBedrockApi支持cohere.embed-english-v3
和cohere.embed-multilingual-v3
模型进行单次和批量嵌入计算。
这里有一个如何以编程方式使用API的简单代码段:
CohereEmbeddingBedrockApi api = new CohereEmbeddingBedrockApi(
CohereEmbeddingModel.COHERE_EMBED_MULTILINGUAL_V1.id(),
EnvironmentVariableCredentialsProvider.create(),
Region.US_EAST_1.id(), new ObjectMapper());
CohereEmbeddingRequest request = new CohereEmbeddingRequest(
List.of("I like to eat apples", "I like to eat oranges"),
CohereEmbeddingRequest.InputType.search_document,
CohereEmbeddingRequest.Truncate.NONE);
CohereEmbeddingResponse response = api.embedding(request);